LeetCode 솔루션 분류
[9/6] 814. Binary Tree Pruning
본문
Medium
3801102Add to ListShareGiven the root
of a binary tree, return the same tree where every subtree (of the given tree) not containing a 1
has been removed.
A subtree of a node node
is node
plus every node that is a descendant of node
.
Example 1:
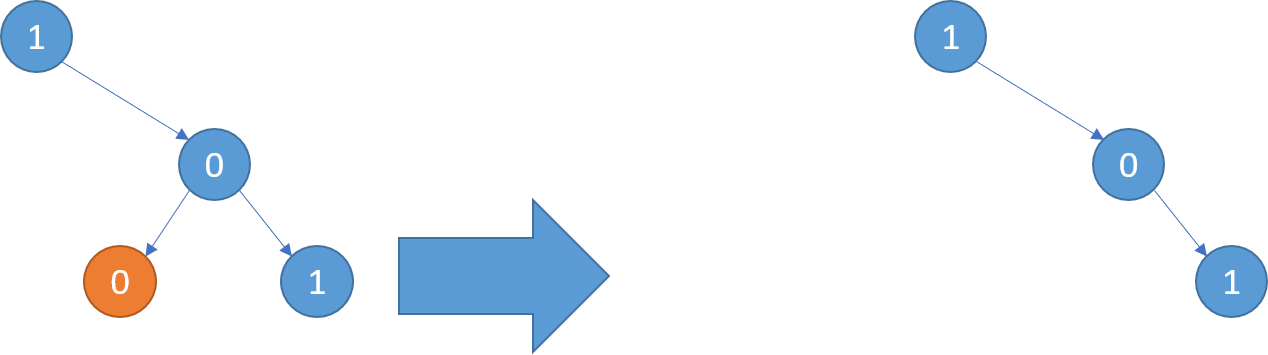
Input: root = [1,null,0,0,1] Output: [1,null,0,null,1] Explanation: Only the red nodes satisfy the property "every subtree not containing a 1". The diagram on the right represents the answer.
Example 2:
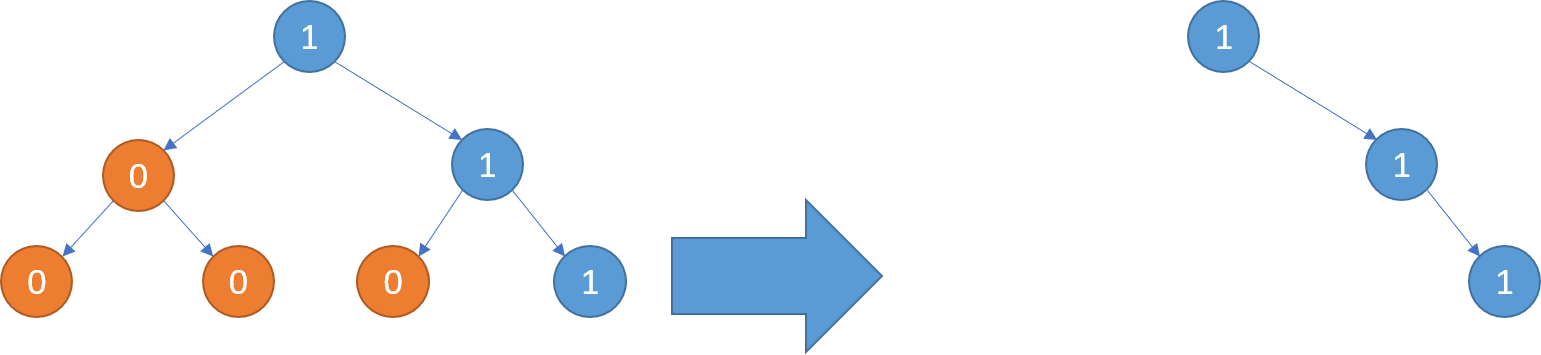
Input: root = [1,0,1,0,0,0,1] Output: [1,null,1,null,1]
Example 3:
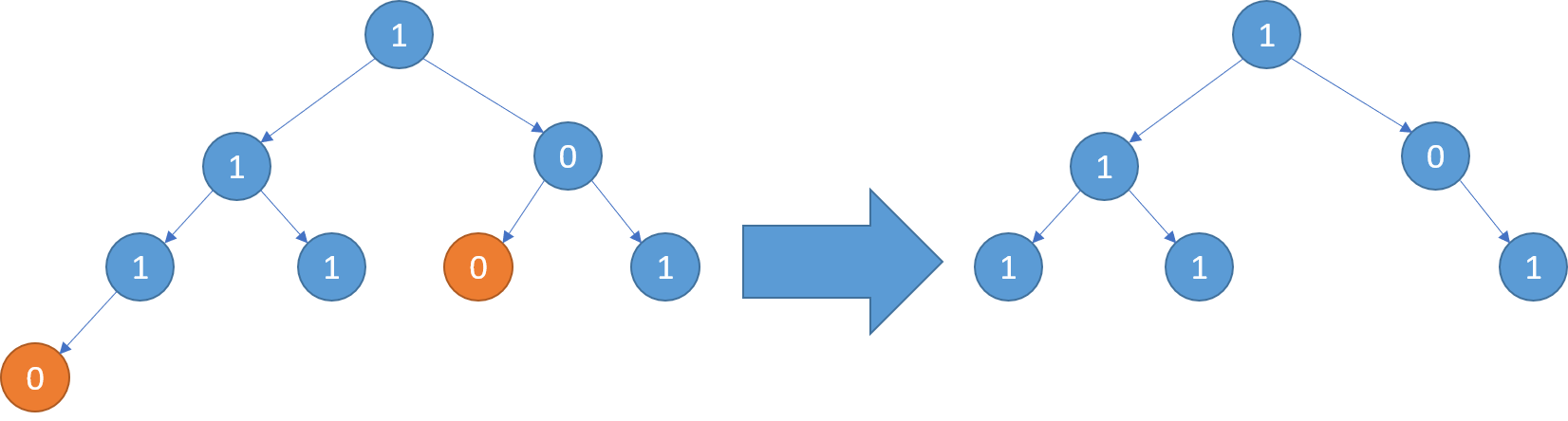
Input: root = [1,1,0,1,1,0,1,0] Output: [1,1,0,1,1,null,1]
Constraints:
- The number of nodes in the tree is in the range
[1, 200]
. Node.val
is either0
or1
.
Accepted
205,596
Submissions
283,200
관련자료
-
링크
댓글 1
학부유학생님의 댓글
- 익명
- 작성일
Runtime: 43 ms, faster than 69.53% of Python3 online submissions for Binary Tree Pruning.
Memory Usage: 14 MB, less than 23.50% of Python3 online submissions for Binary Tree Pruning.
Memory Usage: 14 MB, less than 23.50% of Python3 online submissions for Binary Tree Pruning.
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, val=0, left=None, right=None):
# self.val = val
# self.left = left
# self.right = right
class Solution:
def pruneTree(self, root: Optional[TreeNode]) -> Optional[TreeNode]:
if not root: return None
root.left = self.pruneTree(root.left)
root.right = self.pruneTree(root.right)
if not root.left and not root.right and not root.val: return None
return root