LeetCode 솔루션 분류
[9/7] 606. Construct String from Binary Tree
본문
Easy
22552782Add to ListShareGiven the root
of a binary tree, construct a string consisting of parenthesis and integers from a binary tree with the preorder traversal way, and return it.
Omit all the empty parenthesis pairs that do not affect the one-to-one mapping relationship between the string and the original binary tree.
Example 1:
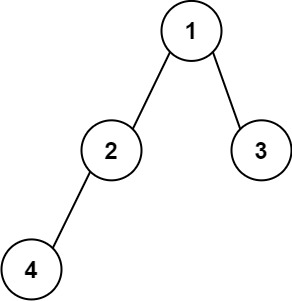
Input: root = [1,2,3,4] Output: "1(2(4))(3)" Explanation: Originally, it needs to be "1(2(4)())(3()())", but you need to omit all the unnecessary empty parenthesis pairs. And it will be "1(2(4))(3)"
Example 2:
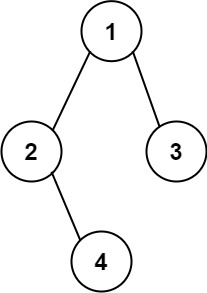
Input: root = [1,2,3,null,4] Output: "1(2()(4))(3)" Explanation: Almost the same as the first example, except we cannot omit the first parenthesis pair to break the one-to-one mapping relationship between the input and the output.
Constraints:
- The number of nodes in the tree is in the range
[1, 104]
. -1000 <= Node.val <= 1000
Accepted
195,076
Submissions
308,524
관련자료
-
링크
댓글 3
KC님의 댓글
- 익명
- 작성일
def tree2str(self, root: TreeNode) -> str:
def preorder(node, parentheses=True):
if node is None:
return ""
l = preorder(node.left)
r = preorder(node.right)
if not l and not r: return str(node.val)
elif l and not r: return str(node.val) + "("+l+")"
# elif not l and r: return str(node.val) + "()" + "("+r+")" # 아래와 같다 !!!
else: return str(node.val) + "("+l+")" + "("+r+")"
return preorder(root)
def preorder(node, parentheses=True):
if node is None:
return ""
l = preorder(node.left)
r = preorder(node.right)
if not l and not r: return str(node.val)
elif l and not r: return str(node.val) + "("+l+")"
# elif not l and r: return str(node.val) + "()" + "("+r+")" # 아래와 같다 !!!
else: return str(node.val) + "("+l+")" + "("+r+")"
return preorder(root)
학부유학생님의 댓글
- 익명
- 작성일
class Solution:
def tree2str(self, root: Optional[TreeNode], string = "") -> str:
string = str(root.val)
if root.left:
string += "(" + self.tree2str(root.left, string) + ")"
if root.right:
if not root.left: string += "()"
string += "(" + self.tree2str(root.right, string) + ")"
return string