LeetCode 솔루션 분류
[12/6] 328. Odd Even Linked List
본문
Given the head
of a singly linked list, group all the nodes with odd indices together followed by the nodes with even indices, and return the reordered list.
The first node is considered odd, and the second node is even, and so on.
Note that the relative order inside both the even and odd groups should remain as it was in the input.
You must solve the problem in O(1)
extra space complexity and O(n)
time complexity.
Example 1:
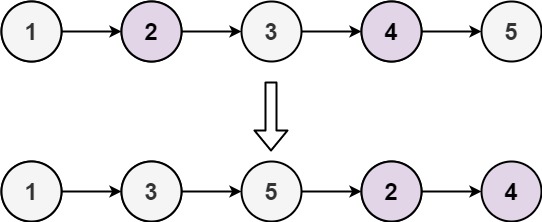
Input: head = [1,2,3,4,5] Output: [1,3,5,2,4]
Example 2:
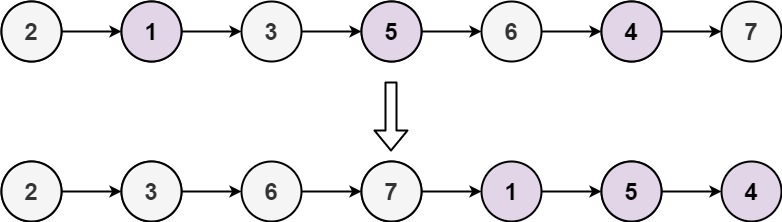
Input: head = [2,1,3,5,6,4,7] Output: [2,3,6,7,1,5,4]
Constraints:
- The number of nodes in the linked list is in the range
[0, 104]
. -106 <= Node.val <= 106
Accepted
663.5K
Submissions
1.1M
Acceptance Rate
61.1%
관련자료
-
링크
댓글 1
학부유학생님의 댓글
- 익명
- 작성일
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, val=0, next=None):
# self.val = val
# self.next = next
class Solution:
def oddEvenList(self, head: Optional[ListNode]) -> Optional[ListNode]:
if not head or not head.next: return head
curr = head
even = curr.next
even_start = even
odd = curr
curr = curr.next.next
while curr and curr.next:
odd.next = curr
curr = curr.next
even.next = curr
curr = curr.next
odd = odd.next
even = even.next
if curr:
odd.next = curr
odd = odd.next
odd.next = even_start
even.next = None
return head