LeetCode 솔루션 분류
[9/4] 987. Vertical Order Traversal of a Binary Tree
본문
Hard
49353892Add to ListShareGiven the root
of a binary tree, calculate the vertical order traversal of the binary tree.
For each node at position (row, col)
, its left and right children will be at positions (row + 1, col - 1)
and (row + 1, col + 1)
respectively. The root of the tree is at (0, 0)
.
The vertical order traversal of a binary tree is a list of top-to-bottom orderings for each column index starting from the leftmost column and ending on the rightmost column. There may be multiple nodes in the same row and same column. In such a case, sort these nodes by their values.
Return the vertical order traversal of the binary tree.
Example 1:
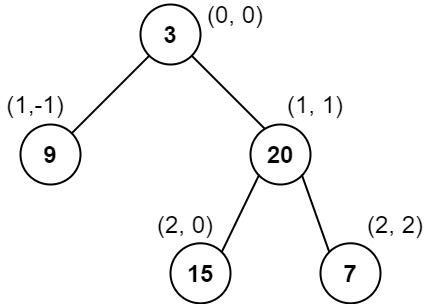
Input: root = [3,9,20,null,null,15,7] Output: [[9],[3,15],[20],[7]] Explanation: Column -1: Only node 9 is in this column. Column 0: Nodes 3 and 15 are in this column in that order from top to bottom. Column 1: Only node 20 is in this column. Column 2: Only node 7 is in this column.
Example 2:
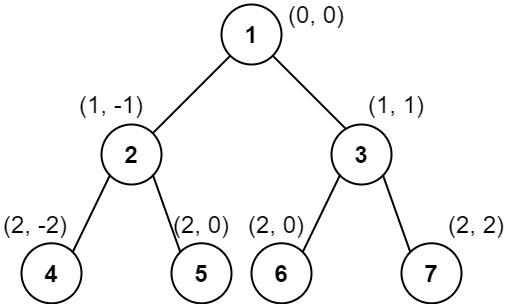
Input: root = [1,2,3,4,5,6,7] Output: [[4],[2],[1,5,6],[3],[7]] Explanation: Column -2: Only node 4 is in this column. Column -1: Only node 2 is in this column. Column 0: Nodes 1, 5, and 6 are in this column. 1 is at the top, so it comes first. 5 and 6 are at the same position (2, 0), so we order them by their value, 5 before 6. Column 1: Only node 3 is in this column. Column 2: Only node 7 is in this column.
Example 3:
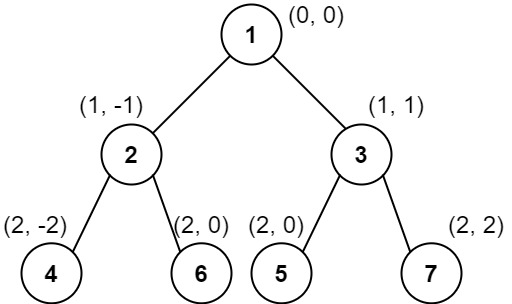
Input: root = [1,2,3,4,6,5,7] Output: [[4],[2],[1,5,6],[3],[7]] Explanation: This case is the exact same as example 2, but with nodes 5 and 6 swapped. Note that the solution remains the same since 5 and 6 are in the same location and should be ordered by their values.
Constraints:
- The number of nodes in the tree is in the range
[1, 1000]
. 0 <= Node.val <= 1000
Accepted
290,064
Submissions
657,120
관련자료
-
링크
댓글 1
학부유학생님의 댓글
- 익명
- 작성일
Runtime: 43 ms, faster than 77.22% of Python3 online submissions for Vertical Order Traversal of a Binary Tree.
Memory Usage: 14.3 MB, less than 28.68% of Python3 online submissions for Vertical Order Traversal of a Binary Tree.
Memory Usage: 14.3 MB, less than 28.68% of Python3 online submissions for Vertical Order Traversal of a Binary Tree.
import collections
class Solution:
def verticalTraversal(self, root: Optional[TreeNode]) -> List[List[int]]:
dic = collections.defaultdict(list)
def dfs(node, vertical_line, level):
if not node: return
dic[vertical_line].append((node.val, level))
dfs(node.left, vertical_line-1, level+1)
dfs(node.right, vertical_line+1, level+1)
dfs(root, 0, 0)
res = []
for key in sorted(dic.keys()):
res.append( [ x[0] for x in sorted(dic[key], key=lambda x: (x[1], x[0])) ] )
return res